I am using .txt files here, but the process should be the same for photographs.
Here is a look at six text files in your_folder.
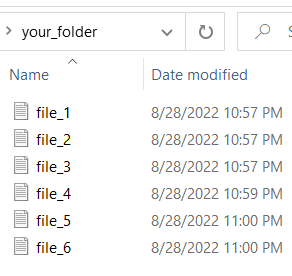
The steps below will read in the file paths, gather timestamp info via file.info
, arrange and group by timestamps to determine which letter to add, and ultimately construct the new filenames.
library(tidyverse)
# read your files
filepaths = list.files('your_folder', full.names = T) # full file paths
filenames = list.files('your_folder') # file names only
# get file info
file_data = lapply(filepaths, file.info) |>
bind_rows() |>
# add a column name for filename
bind_cols(
tibble(filename = filenames)
) |>
rownames_to_column('filepath_original') |>
arrange(mtime) |>
# group by files with the same timestamp (mtime)
# and generate the associated letter (where appropriate)
group_by(mtime) |>
mutate(max_rows = max(row_number())) |>
mutate(letter = ifelse(max_rows > 1,
letters[row_number()],
'')
) |>
ungroup() |>
# clean up the time string
mutate(time = str_replace_all(mtime, '-', ''),
time = str_replace_all(time, ':', ''),
time = str_replace(time, ' ', '_')) |>
# construct the new file path
mutate(filename_new = paste0('ProjectName_', time, letter)) |>
mutate(filepath_new = str_replace(filepath_original, filename, ''),
filepath_new = paste0(filepath_new, filename_new, '.txt')
) |>
select(filepath_original, filepath_new)
Executing this code produces...
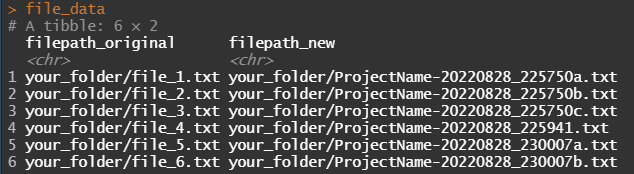
Finally, use file.rename()
to rename the files in the folder.
# rename in folder
file.rename(from = file_data$filepath_original,
to = file_data$filepath_new)
This ultimately results in the six newly named files below.
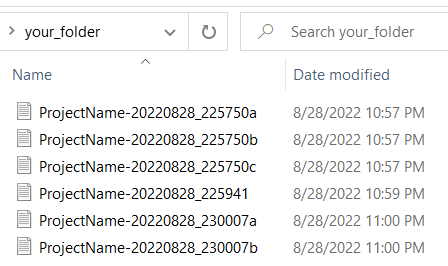