I just have noticed that polygons are not where they should be on the stamen map, there is a small offset. I have found a solution and explanation for this problem on this github issue, here is the example with the solution.
library(tidyverse)
library(ggmap)
library(sf)
scores <- data.frame(stringsAsFactors = FALSE,
NAME_3 = c("Berlin", "Hannover", "Dresden", "Frankfurt am Main", "Stuttgart"),
score = c(80, 70, 90, 100, 20))
url_regions <- "https://biogeo.ucdavis.edu/data/gadm3.6/Rsf/gadm36_DEU_3_sf.rds"
scored_regions <- readRDS(url(url_regions)) %>%
filter(NAME_3 %in% scores$NAME_3) %>%
full_join(scores)
germany_stamen <- get_stamenmap(bbox = c(left = 5,
bottom = 47,
right = 15,
top = 55),
zoom = 6,
maptype = "toner")
# Define a function to fix the bbox to be in EPSG:3857
ggmap_bbox <- function(map) {
if (!inherits(map, "ggmap")) stop("map must be a ggmap object")
# Extract the bounding box (in lat/lon) from the ggmap to a numeric vector,
# and set the names to what sf::st_bbox expects:
map_bbox <- setNames(unlist(attr(map, "bb")),
c("ymin", "xmin", "ymax", "xmax"))
# Coonvert the bbox to an sf polygon, transform it to 3857,
# and convert back to a bbox (convoluted, but it works)
bbox_3857 <- st_bbox(st_transform(st_as_sfc(st_bbox(map_bbox, crs = 4326)), 3857))
# Overwrite the bbox of the ggmap object with the transformed coordinates
attr(map, "bb")$ll.lat <- bbox_3857["ymin"]
attr(map, "bb")$ll.lon <- bbox_3857["xmin"]
attr(map, "bb")$ur.lat <- bbox_3857["ymax"]
attr(map, "bb")$ur.lon <- bbox_3857["xmax"]
map
}
germany_stamen_crs_3857 <- ggmap_bbox(germany_stamen)
ggmap(germany_stamen_crs_3857) +
coord_sf(crs = st_crs(3857)) +
geom_sf(data = st_transform(scored_regions, 3857),
aes(fill = score),
inherit.aes = FALSE,
alpha = 0.7) +
scale_fill_gradient(low = "red", high = "green") +
labs(title = "Germany Scores",
fill = "Scores") +
theme_minimal() +
theme(plot.title = element_text(hjust = 0.5, size = 20),
axis.title = element_blank(),
plot.background = element_blank(),
legend.position = "bottom",
plot.margin = margin(5, 0, 5, 0))
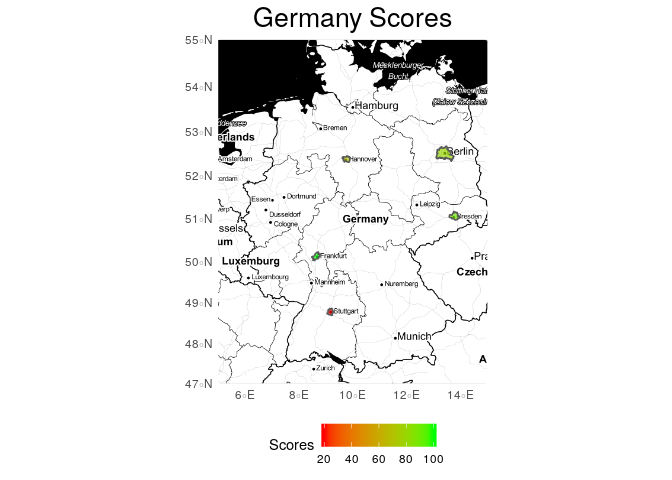