Here are a couple of ways I know of to preserve hyperlinks when writing to .csv/.xlsx
Hacky Method
Excel has a HYPERLINK
function that has the format =HYPERLINK(LINK, TEXT)
so we can create a column with exactly this text. Then when you open the .csv file with Excel, the formula gets parsed. Obvious downside is that this is an Excel-specific function (as far as I know) so any other .csv viewer will probably fail to render the link.
# Imports
library(dplyr)
#>
#> Attaching package: 'dplyr'
#> The following objects are masked from 'package:stats':
#>
#> filter, lag
#> The following objects are masked from 'package:base':
#>
#> intersect, setdiff, setequal, union
library(tibble)
library(glue)
#>
#> Attaching package: 'glue'
#> The following object is masked from 'package:dplyr':
#>
#> collapse
# Some mock data
test_data <- tribble(
~id, ~link,
"Example link #1", "https://google.ca",
"Example link #2", "https://forum.posit.co"
) %>%
mutate(
link = glue(
'=HYPERLINK("{link}", "{id}")'
)
)
write.csv(test_data, "test.csv", row.names = FALSE)
Created on 2019-08-19 by the reprex package (v0.3.0)
Output:
Using openxlsx
A cleaner solution is to use openxlsx
. We can set the link column's class to hyperlink and openxlsx
will respect that class when writing.
# Imports
library(dplyr)
#>
#> Attaching package: 'dplyr'
#> The following objects are masked from 'package:stats':
#>
#> filter, lag
#> The following objects are masked from 'package:base':
#>
#> intersect, setdiff, setequal, union
library(tibble)
library(openxlsx)
# Some mock data
test_data <- tribble(
~id, ~link,
"Example link #1", "https://google.ca",
"Example link #2", "https://forum.posit.co"
)
class(test_data$link) <- "hyperlink"
write.xlsx(
test_data,
"test_out.xlsx"
)
#> Note: zip::zip() is deprecated, please use zip::zipr() instead
Created on 2019-08-19 by the reprex package (v0.3.0)
Output:
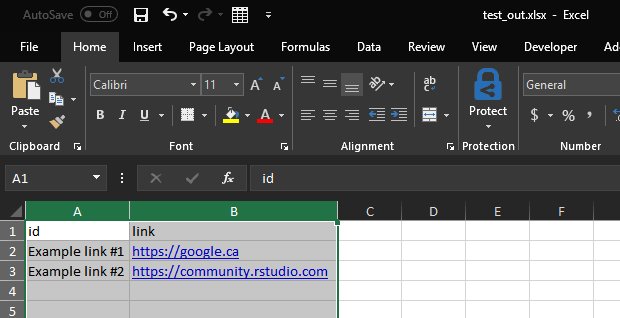