I’m not sure if I understand what you want to do, but I would recommend using sf
and tidyverse
packages to explode or combine coordinates.
I don’t have your original data, but you should be able to use read_sf()
to load them.
library(tidyverse)
library(sf)
# You should be able to use the following line
#read_sf("ljz_network.gdb",layer = "Export_Output")
# I'll have to make data, hopefully their structure matches what you have
lines <- st_sf(
id = 1:2,
geometry = st_as_sfc(c(
"LINESTRING(0 0, 2 3, 1 4, 0 3)",
"LINESTRING(0 0, -2 3, -1 4, 0 3)"))
)
lines
#> Simple feature collection with 2 features and 1 field
#> geometry type: LINESTRING
#> dimension: XY
#> bbox: xmin: -2 ymin: 0 xmax: 2 ymax: 4
#> CRS: NA
#> id geometry
#> 1 1 LINESTRING (0 0, 2 3, 1 4, ...
#> 2 2 LINESTRING (0 0, -2 3, -1 4...
plot(lines$geometry)
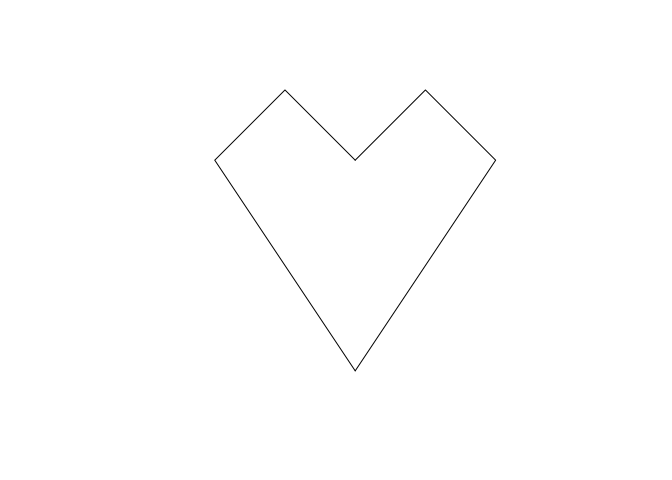
# explode lines
points <- lines %>%
rowwise() %>%
mutate(
coordinates = list(st_coordinates(geometry)),
) %>%
unnest(coordinates) %>%
group_by(id) %>%
mutate(
x = coordinates[, 1],
y = coordinates[, 2],
l1 = coordinates[, 3],
# MULTILINESTRINGS would have an additional column for each part
order = row_number(),
coordinates = NULL # drop the raw coordinate column
) %>%
ungroup()
# make points
points <- points %>%
rowwise() %>%
mutate(
geometry_point = st_sfc(list(st_point(c(x, y))))
)
points
#> Simple feature collection with 8 features and 5 fields
#> Active geometry column: geometry
#> geometry type: LINESTRING
#> dimension: XY
#> bbox: xmin: -2 ymin: 0 xmax: 2 ymax: 4
#> CRS: NA
#> # A tibble: 8 x 7
#> # Rowwise:
#> id geometry x y l1 order geometry_point
#> * <int> <LINESTRING> <dbl> <dbl> <dbl> <int> <POINT>
#> 1 1 (0 0, 2 3, 1 4, 0 3) 0 0 1 1 (0 0)
#> 2 1 (0 0, 2 3, 1 4, 0 3) 2 3 1 2 (2 3)
#> 3 1 (0 0, 2 3, 1 4, 0 3) 1 4 1 3 (1 4)
#> 4 1 (0 0, 2 3, 1 4, 0 3) 0 3 1 4 (0 3)
#> 5 2 (0 0, -2 3, -1 4, 0 3) 0 0 1 1 (0 0)
#> 6 2 (0 0, -2 3, -1 4, 0 3) -2 3 1 2 (-2 3)
#> 7 2 (0 0, -2 3, -1 4, 0 3) -1 4 1 3 (-1 4)
#> 8 2 (0 0, -2 3, -1 4, 0 3) 0 3 1 4 (0 3)
plot(points$geometry_point)
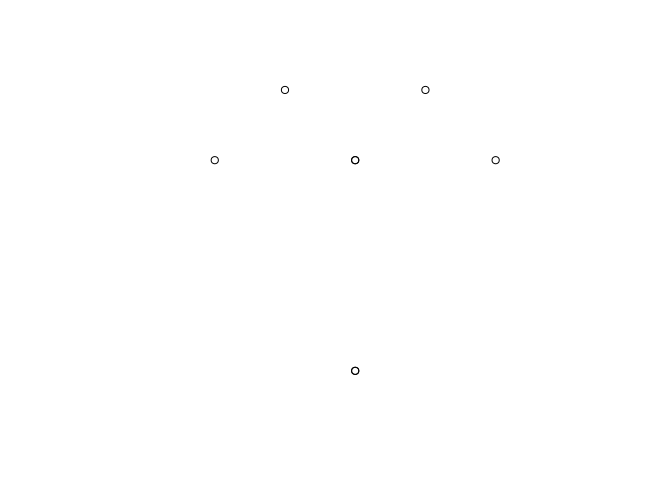
# make lines from points
points %>%
arrange(l1) %>%
group_by(id) %>% # could add part if doing multilinestring
summarise(
geometry_line = st_cast(st_combine(geometry_point), "LINESTRING")
)
#> `summarise()` ungrouping output (override with `.groups` argument)
#> Simple feature collection with 2 features and 1 field
#> geometry type: LINESTRING
#> dimension: XY
#> bbox: xmin: -2 ymin: 0 xmax: 2 ymax: 4
#> CRS: NA
#> # A tibble: 2 x 2
#> id geometry_line
#> <int> <LINESTRING>
#> 1 1 (0 0, 2 3, 1 4, 0 3)
#> 2 2 (0 0, -2 3, -1 4, 0 3)
Created on 2021-09-22 by the reprex package (v0.3.0)